We are having a few performance issues when calling middle tier web services repeatedly at very close intervals and I wanted to raise this to see if any other peeps out there have stumbled on this before we use one of our valuable Microsoft support incidents. I have developed a very simple web service Page to demonstrate this in DynamicsNAV2009R2 see function below:
//Codeunit exposing WebService function
FncAnswerToUniverse() : Integer
// Called by ASP.NET to test latency communication with middle tier
EXIT(42)
using System;
using System.Collections.Generic;
using System.Text;
namespace PerformanceChecker
{
// Import newly generated Web service proxy.
using DemoCallWSCustomerPage.WebService;
class Program
{
static void Main(string[] args)
{
int IntTheAnswerIs = 0;
// Create instance of service and set credentials.
SvcSTPGlobalFunctions GlobalFunctions = new SvcSTPGlobalFunctions();
GlobalFunctions.Url = "http://SOV-NAV-T-APP2:7047/DynamicsNAV/WS/Kelway2009ST/Codeunit/SvcSTPGlobalFunctions";
GlobalFunctions.UseDefaultCredentials = true;
int numberOfIterations = 50;
for (int i = 0; i < numberOfIterations; i++)
{
DateTime start = DateTime.Now;
IntTheAnswerIs = GlobalFunctions.FncAnswerToUniverse();
DateTime end = DateTime.Now;
TimeSpan runTime = end - start;
Console.WriteLine("The answer to the universe is: {0} and it took {1} m/s to compute!", IntTheAnswerIs, runTime.Milliseconds.ToString());
//Comment out and run full speed and compare time to compute
System.Threading.Thread.Sleep(300);
}
Console.WriteLine("Press [ENTER] to exit program!");
Console.ReadLine();
}
}
}
When we repeatedly call this from an ASP.NET test harness we get timings of around 200ms for each round trip to the web service (Running on clients mega servers)
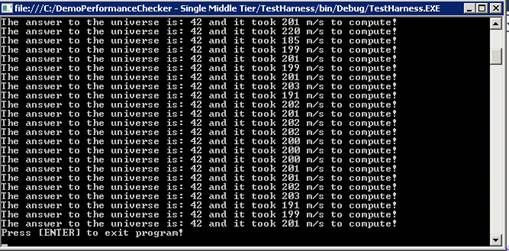
If we place a delay of 300m/s between each call (Uncomment line in sample attached) to the web service function we get responses round trip responses of arounf 8-10ms
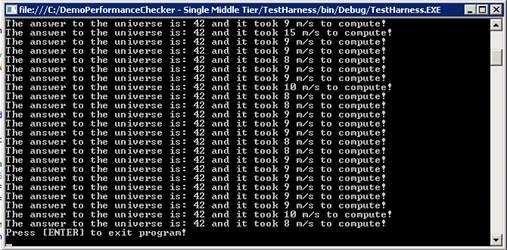
It seems to indicate there is something within the middle tier service that is throttling responses back one it has replied to a request.
We have also written a test harness to send the request in sequence to 4 separate middle tiers services. This handles the load a lot better, but we still get the 200ms delay on each middle tier service before we can re-use it (see tier 0 timings) but because the requests are spread across multiple middle tiers it handles the load better. See screen shot below from our development server:
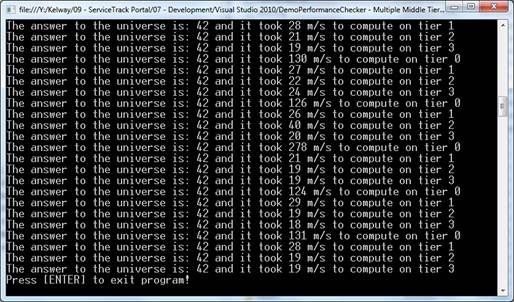
Has anyone else experienced performance issues with Webservice calls like this??? Any help/ideas would be appreciated.
Thank you!
Comments
If you find something, please post it here, cause i'm also doing some work about WS
"Never memorize what you can easily find in a book".....Or Mibuso
My Blog
Bergamo?? I was in Northers Italy (Passo del Bocco) last month for Giro del Italia & Mille Miglia !! Absoulutly Fantastic
Independent Consultant/Developer
blog: https://dynamicsuser.net/nav/b/ara3n
I find it quite amazing it is exaclty 200ms!!! If I put a sleep of 100ms it still takes 100ms for web service call adding upto a total of 200ms give or take the odd ms!!!!
Now that rashed tell it, i've also heard about slowness due to delegation one year ago in MS italy, but my memory is ethereal
I'm glad you liked it! One day i hope to travel in US and feed you back as well
"Never memorize what you can easily find in a book".....Or Mibuso
My Blog
Our portal login procedure executes 4 webservice calls, this is taking around 1000ms because of this issue and we need to get this as fast as possible. Data binding to Telerik controls and page load are taking 50-100ms.
Thank you again
Scott
MVP - Dynamics NAV
My BLOG
NAVERTICA a.s.
"Never memorize what you can easily find in a book".....Or Mibuso
My Blog
I tried calling two different Webservices as detailed in ur Pseudo (This is what our portal does FncCheckCompany, FncLogin, FncValidateCustomerDetails, so exepected the same issue) and yes I receive the same 200ms delay between each call
If I run the test application in multiple times in separate threads I still get the delay but there is no drop in speed even with 10-20 copys running...
Antivirus... I have disabled Symantic on both tiers but difficult to remove without speaking to the gods... It's not made any difference disabling it...
My thought now is as suggested to move away from Kerberos and Delegation if this is possible???? I'm going to look into 'WebServicesUseNTLMAuthentication' setting in the CustomSetting.Config for the middle tier. Our web portal has it's own security engine and there is no need for pass through authentication/delegation and all the overheads.
Cheers
If we add dummy parameters (with values) to the exposed web service function FncAnswerToUniverse(TxtPadding1 : Text[1024];TxtPadding2 : Text[1024]) so that the SOAP packet the ASP.NET client generates is > 1460 character the response is immediate. ie. around 15ms per request instead of 200ms!!!!
There are registery hacks for this on the internet but if we implement these it will effect the speed of large multi-packet responses (Streaming order history data). We are unsure what to do at this stage. Ideally there needs to be some why to disable the Nagle algorithm for each server request. Reading RFC's for WinSock this protocol was modified to allow this.
I now wonder if there is some way the middle tier can be insturcted to not use the Nagle algorithm in certain requests... If anyone out there has encountered this it would be appreciated...
you should mark this as Good posting, and talk with ms about it..
i don't know anything about "Nagle algorithm RFC 896", i have to study it, i'm really curious now.
thanks for sharing! :thumbsup:
"Never memorize what you can easily find in a book".....Or Mibuso
My Blog
By the way for those who havent read 'Hitchhiker's Guide to the Galaxy'... http://en.wikipedia.org/wiki/Phrases_from_The_Hitchhiker%27s_Guide_to_the_Galaxy#Answer_to_the_Ultimate_Question_of_Life.2C_the_Universe.2C_and_Everything_.2842.29
Thanks again for for your comments thus far and if anyone else has experience of this issue it would be good to know...
At the moment I don't have access to a test setup, but you could try these two things:
1) Changing the client:
You can create your own proxy around the generated webservice bindings.
For example like this class:
2) Changing the server:
In the Microsoft.Dynamics.NAV.Server.exe.config file, you could try to add the following
Best Regards
Kenneth
Editing the .config file and restarting the middle tier did not make a difference BUT settiing the two properties on the ServicePointManager prior to the first connection worked \:D/ !!!!!
No news from Microsoft as yet, I was told it's holiday time and not to expect a quick response... We've currently edited all Webservice calls to add two dummy text parameters of 700 characters and changed all Reads to ReadMultiple so that we can pass a filter string that is repeated many times so that it increases the packet size to over 1460 characters!! It will take us some time to change all these back and test but excellent news as it works on the test harness!
Thank you again
I will make sure to try and implement it in one of our test environments when I am back in the office in a few days, to verify that it also works there.
If only I had a blog.... :-)
I know this thread has been inactive for some time, but we found good use of your advise regarding disabling the Nagle algorithm.
We are getting data fore our webshop from a NAV2013 and experienced some slow response times from the web services.
So we started to investigate on the Nagle issue and got it to work thanks to your super advice.
Our test code just calls "DisableNagleAlgorithm" and voila - the response is now down to 25-35 ms instead of 150-170ms
But we still have some issues:
- the first call to the web service is still very slow, like 700 ms. And if the web server is inactive for some time the high "first call" response time is seen again.
- the other issue we have is when we request data from a oData web service (using a NAV 2013 page object). We do this using LINQ, but can't seem to get to disable the Nagle algorithm.
If there is anyone who have solved these issue or just have some ideas on how to fix this, we'll be very glad to get some feedback.
Best regards,
Allan Houmark Persson
Allan Houmark Persson
Great to know that you had use of it :-)
I have not yet tried to work with the ODATA services, but I have had experience with the other issue. Quite simply, this happens to all .NET-based websites in the process of saving resources on the webserver.
Microsoft created a tool for IIS that can be used to solve the issue. Depending on which version of Windows Server you are running the tool has different names. The latest is IIS Auto-Start. Read more about it here: https://www.simple-talk.com/blogs/2013/ ... t-feature/
The NAV webservice is affected by the same issue, but the MS tool cannot be used outside of IIS. If your website calls the NAV webservice on any request the IIS Auto-Start will indirectly solve this as well. If not, you can create a simple scheduled job to keep the NAV webservice alive.
Hope that helps.